Exercise: A First Python Program running on the Android Mobile Phone
We are using the language Python for these exercises. It is a free language that is used by many companies and academic institutions.
We teach Python in one of our second year courses and we also make use of the Android phones in second and third year courses where we look at how to develop Java programs that make use of communications (for example, a program running on an Android phone collects location data that is used to co-ordinate with other programs running on other phones or computers connected via the Internet).
Python runs on a whole range of computers and operating systems (Macs with OS X, PCs with Windows or Linux and mobile devices). Much of the code you write to run on one computer system can be reused on another with minimal change.
Python can be used to create sophisticated programs with complex interfaces but also it is commonly used because it is considered to be easy to try out ideas quickly by using Python to "glue together" existing functions. Once the ideas have been tried out, more sophisticated and reliable versions can be written either in Python or other languages such as Java.
The purpose of these exercises is to give you a flavour of programming and programming modern mobile devices that have access to other computers via the Internet.
The best way to do it is to type in code that I show you to see what happens. Play around with it and make changes. The worst that can happen is that it won't work. When I type in code it will be formatted like this:
print('Hello')
print('Android')
print('from')
print('XXXXX')
That's so it is easy to distinguish from the other text. If you're reading this on the web, you'll notice the code is in color -- that's just to make it stand out, and to make the different parts of the code stand out from each other. The code you enter will probably not be colored, or the colors may be different, but it won't affect the code as long as you enter it the same way as it's printed here.
You will also notice the line numbers on each line. When typing in code, you should ignore these lines. They are only there for reference so we can refer to line X or line Y when discussing the code with other programmers.
On a PC, you could start the Python Interpreter (a program that can read Python scripts or programs and follow the instructions contained within them) and type in the commands above. You could actually do the same on the android phones but using the onscreen keyboard is difficult and the screen is small so we have other ways to tackle entering the program.
For example:
- Create the program on the PC using a program called gedit (it is a text editor, almost any would do here).
- Push the program to the phone using a program called push.
- Run the program on the phone using a program called SL4A.
- Check if it runs okay, if it doesn't you have to work out why and edit the program before sending it across again.
We will do this for the GPS Exercise so you don't have to type in a longer program and because the focus of that exercise is on the GPS capabilities of the phone rather than programming.
Loading and Running Your First Script
1. Start a Terminal on the PC. A Terminal is a command line interface to the computer. On our computers it looks like this and is found at the bottom of the screen. Just click on it once to start it.

You should see a prompt waiting for you type a command, something like this:
brer-fox: [~] %
Where
brer-fox is the name of the computer you are using and
[~] means any files you create will be stored in your own user's file space.
2. Now type the following to start a text editor called gedit and create the script file
cyber-helloworld.py.
gedit cyber-helloworld.py
The text editor will also apply colour highlighting. A bit like a human language, different words have different functions and are coloured accordingly to help the programmer spot when they have made a mistake.
3. Type the following script into the text editor. Replace
XXXXX with a name, it can be anything as long as it is between the quote (') symbols. With anything outside the quote symbols, be careful to make it exactly the same because computers are unforgiving and simple mistakes such as a space between letters in a word will cause it to complain and stop at that point in script (that said, you can't break anything so these "syntax" errors are easily fixed).
print('Hello')
print('Android')
print('from')
print('XXXXX')
Save the script (
File -> Save, this means look for the menu File and the option Save within the menu).and exit the editor (
File -> Quit).
4. Now we are going to copy the script to the phone where it will run. In the
Terminal type:
push cyber-helloworld.py
You should see something like the following (you may not see the first two lines, this happens only once generally and is to do with establishing communication with the phone, the third one is the one that indicates that the copying took place):
* daemon not running. starting it now on port 5037 *
* daemon started successfully *
0 KB/s (72 bytes in 0.335s)
5. Finally we will run the script on the phone. Find the program SL4A and tap it. You should see a list of scripts (probably a bit different to below but still a list of scripts).
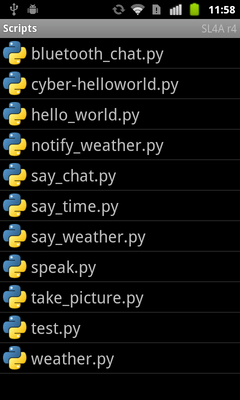
Select *cyber-helloworld.py" by tapping on it to show the quickmenu. You should see:
Start the script by choosing the Run (with Terminal) option (highlighted in red below) -- the wheel runs it without the Terminal and the pencil allows you to edit the script directly on the phone.
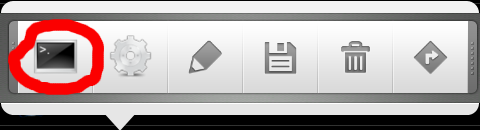
This will bring up a Terminal on the phone where you can see the result of running the program (use the volume controls on the left side of the phone to enlarge the size of the output).
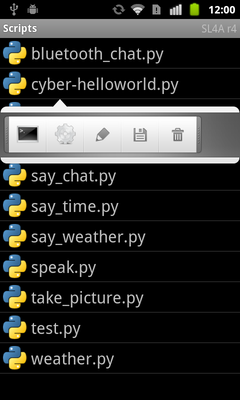
You should see something like the following (I replaced XXXXX with Ian) after the program runs, finishes (process terminates) and an exit dialog is displayed.
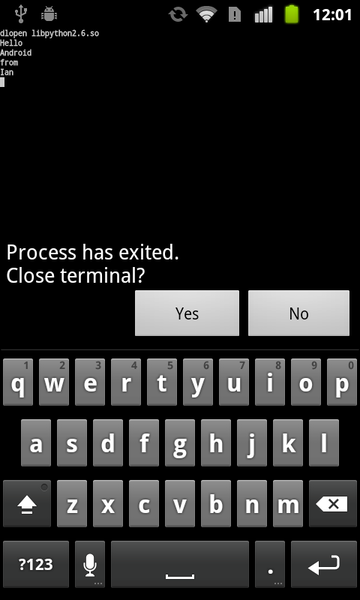
Choose
YES to close the program (the data won't be lost because it is being stored on the phone's SDCARD).
What is Going on Here
The computer executes one line at a time in order. In this case it is printing to the Terminal. The computer keeps looking at each line, follows the command and then goes on to the next line. The computer keeps running commands until it reaches the end of the program. Should you want to extend what is printed just add more print commands to the program.
Now is probably a good time to give you a bit of an explanation of what is happening - and a little bit of programming terminology.
What we were doing above was using a command called print. The print command is followed by one or more arguments. So in this example
print('Hello')
there is one argument, which is 'Hello'. Note that this argument is a group of characters enclosed in single quotes (') or double quotes ("). This is commonly referred to as a string of characters, or string, for short. Another example of a string is 'Hello, World'. You are allowed to use spaces within the quotes. Note also that you can also print numbers which do not need to be enclosed in single quotes (') or double quotes (").
A command and its arguments are collectively referred to as a statement, so
print('Hello')
is an example of a statement.
That's probably more than enough terminology for now..
Troubleshooting
Maybe you didn't see the expected result? Don't worry. Things usually go wrong with programs. Fixing them is called debugging. So it is useful to know some typical errors and what it means. These errors shown here are generally fixed by going back and checking you typed in exactly what was specified!
Syntax errors
An example is shown below. Here "print" has a space between "p" and "rint". It also tells you that the error appears to be on line 1.
Note that sometimes the error is on the line before, errors on one line can cause another on lines further down in the program!
Name errors
An example is shown below. Here "print" has been called "pr" and the computer does not know what "pr" could means. Here I just didn't copy what was specified but sometimes it means you are trying to do something that the computer doesn't know how to yet but could be setup to understand.
Scanning errors
An example is shown below. In this case there is a missing ending single quote ('). Add the missing quote. Quotes must always balance, a bit like brackets in algebra. Otherwise the computer can't work out where the string ends and it "scans" looking for it until it falls off the end of the line (EOL = end of line).